Latent Consistency Models on Your Apple Silicon MacBook Pro in seconds
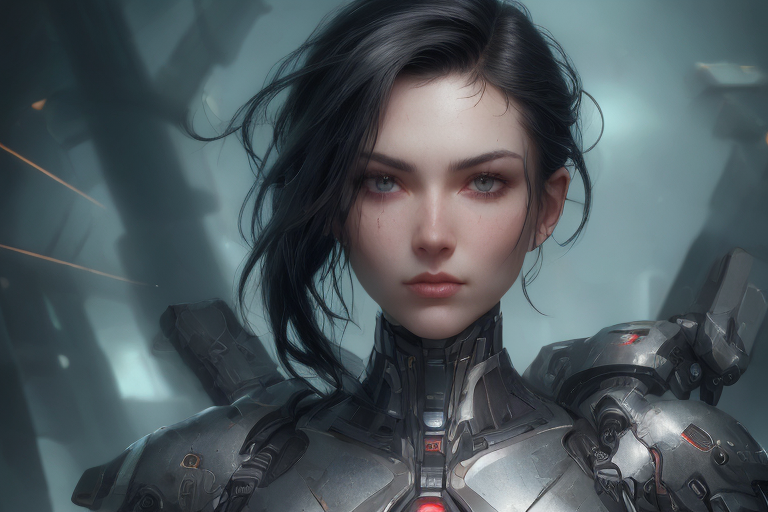
The code provided below illustrates the utilization of Latent Consistency Models for efficient high-resolution image generation on an Apple Silicon MacBook Pro. It commences by configuring a diffusion pipeline and establishing crucial parameters, including image dimensions and inference steps. A thoughtfully crafted prompt specifies the desired image, be it a portrait or a cyborg, with the flexibility to control the number of inference steps. The code optimizes the process for GPU memory and performance, resulting in the generation of multiple images based on the specified prompt. These resultant images are preserved for subsequent analysis. Through a blend of specific configurations and the capabilities of Apple Silicon, this code serves as a demonstration of the potential of Latent Consistency Models in the realm of creative image synthesis.
We conducted image generation on an M1 MacBook Pro using four inference steps, with completion times ranging from 4 to 25 seconds. While the initial model requires loading into RAM, subsequent generations demonstrate increased efficiency.
The linked repository serves as the primary resource for examples, from which we extracted portions of code and developed a concise Python script. Additionally, we have included comments to facilitate understanding of the code's inner workings.
Example Code:
from diffusers import DiffusionPipeline
import os
import random
import time
import torch
import uuid
# Start the timer
start_time = time.time()
# Max seed setting
MAX_SEED=2147483647
# Setup the pipe
pipe = DiffusionPipeline.from_pretrained(
"SimianLuo/LCM_Dreamshaper_v7",
custom_pipeline="latent_consistency_txt2img",
custom_revision="main",
revision="fb9c5d"
)
# Width and height setting
width=512
height=512
# To save GPU memory, torch.float16 can be used, but it may compromise image quality.
pipe.to(
torch_device="mps",
torch_dtype=torch.float32
)
# Image save directory
root_path = './images/'
def save_image(img, root_path='./'):
""" Create a uuid, and save PNG image """
unique_name = str(uuid.uuid4()) + '.png'
unique_name = os.path.join(root_path, unique_name)
img.save(unique_name)
return unique_name
# Write a great prompt
prompt = "portrait photo, a beautiful cyborg with black hair, 8k"
# Can be set to 1~50 steps. LCM support fast inference even <= 4 steps. Recommend: 1~8 steps.
num_inference_steps = 4
# Setup your CFG scale
guidance_scale = 8.0
# How many images to output
num_images=2
# Just a random seed on every run
seed = random.randint(0, MAX_SEED)
print(f"Seed: {seed}")
torch.manual_seed(seed)
# Magic time, generate the image
images = pipe(
prompt=prompt,
width=width,
height=height,
num_inference_steps=num_inference_steps,
num_images_per_prompt=num_images,
guidance_scale=guidance_scale,
lcm_origin_steps=50,
output_type="pil").images
# Save the list of images
for image in images:
img_saved = save_image(img=image, root_path=root_path)
print(img_saved)
# Print out the timing
print(time.time() - start_time)
Example Output:
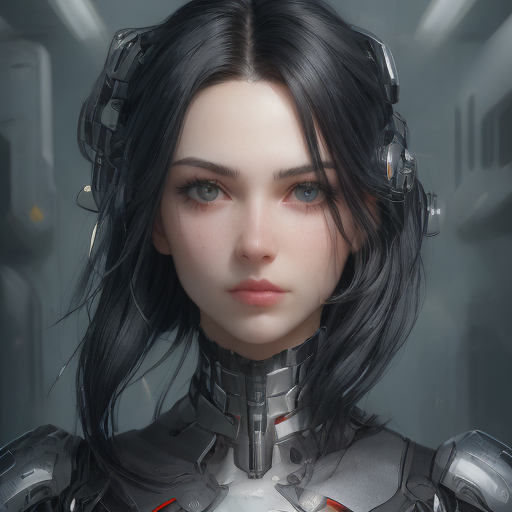