How to post images from Stable Diffusion to Discord
Adding a post to Discord function to my Stable Diffusion install
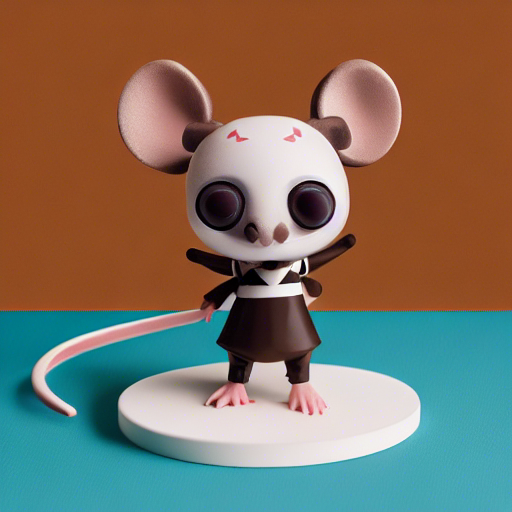
I wanted to post my Stable Diffusion creations to Discord to
keep track of them over time. Since SD is just Python under
the hood, I ended up modifying my install.
Here is how I did it.
- Get a Discord webhook for a channel to post to
- Create a Python script to post data to that webhook
- Integrate the new script with text2img and img2img scripts
Get a Discord webhook for a channel to post to
Create a new channel on your Discord server
Right click and edit the channel
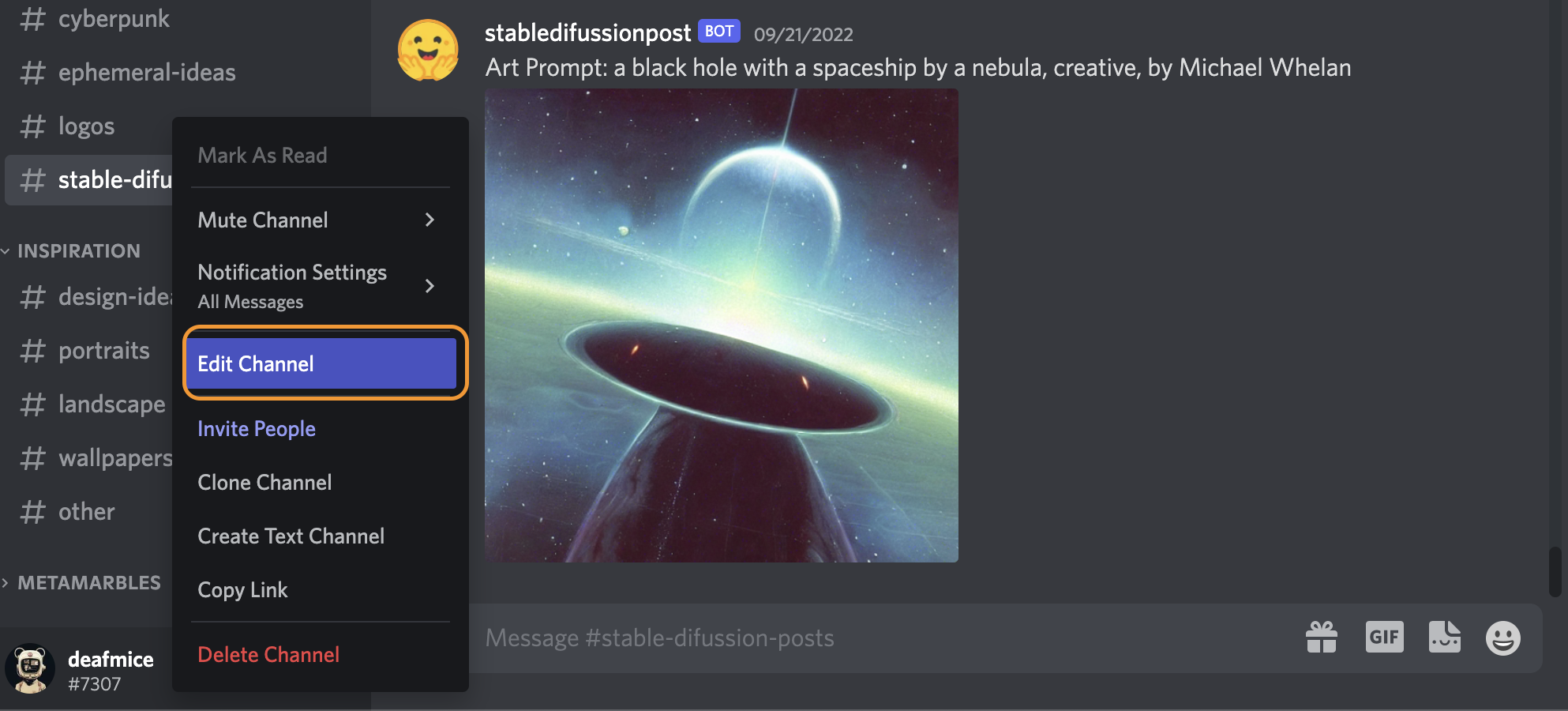
Click on Integrations, and then view wehbooks
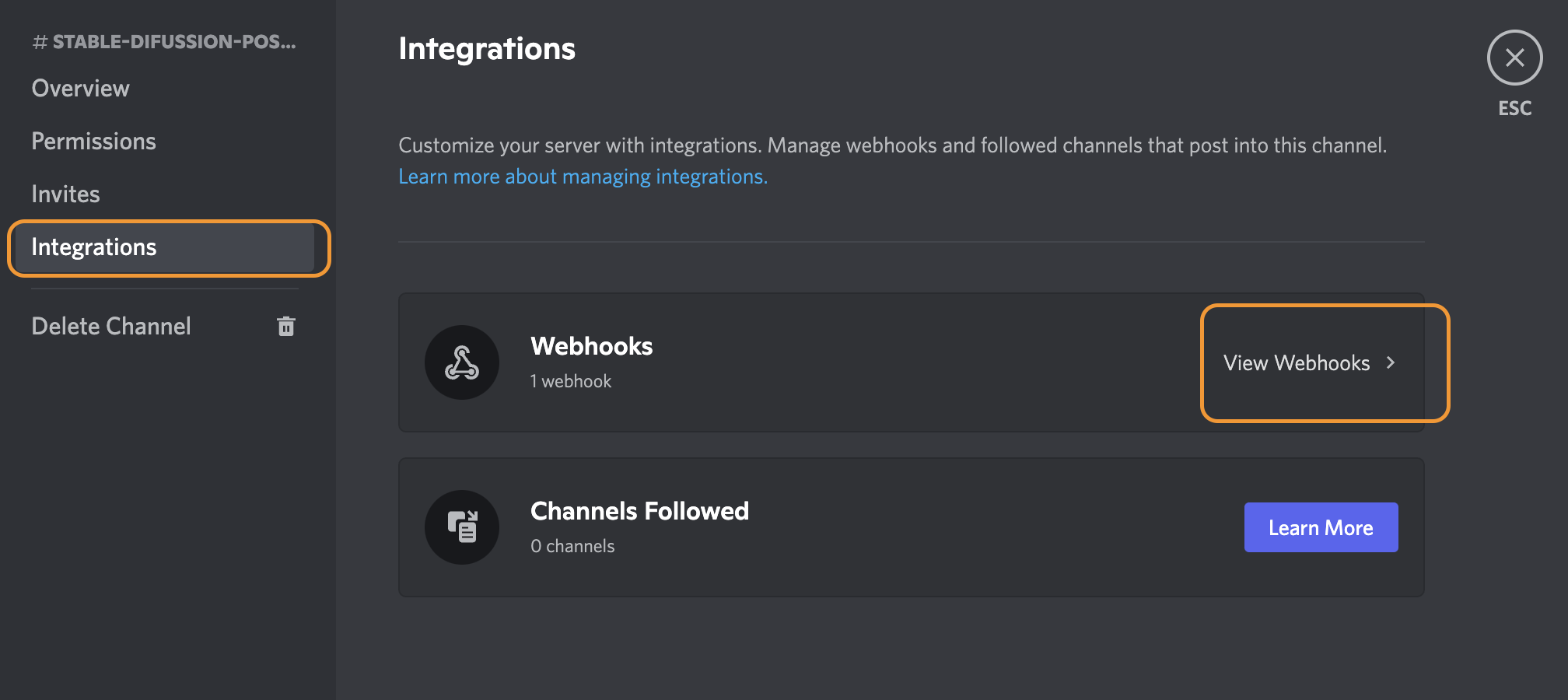
Click new webhook, name it something fancy, add a icon, and then copy the webhook URL
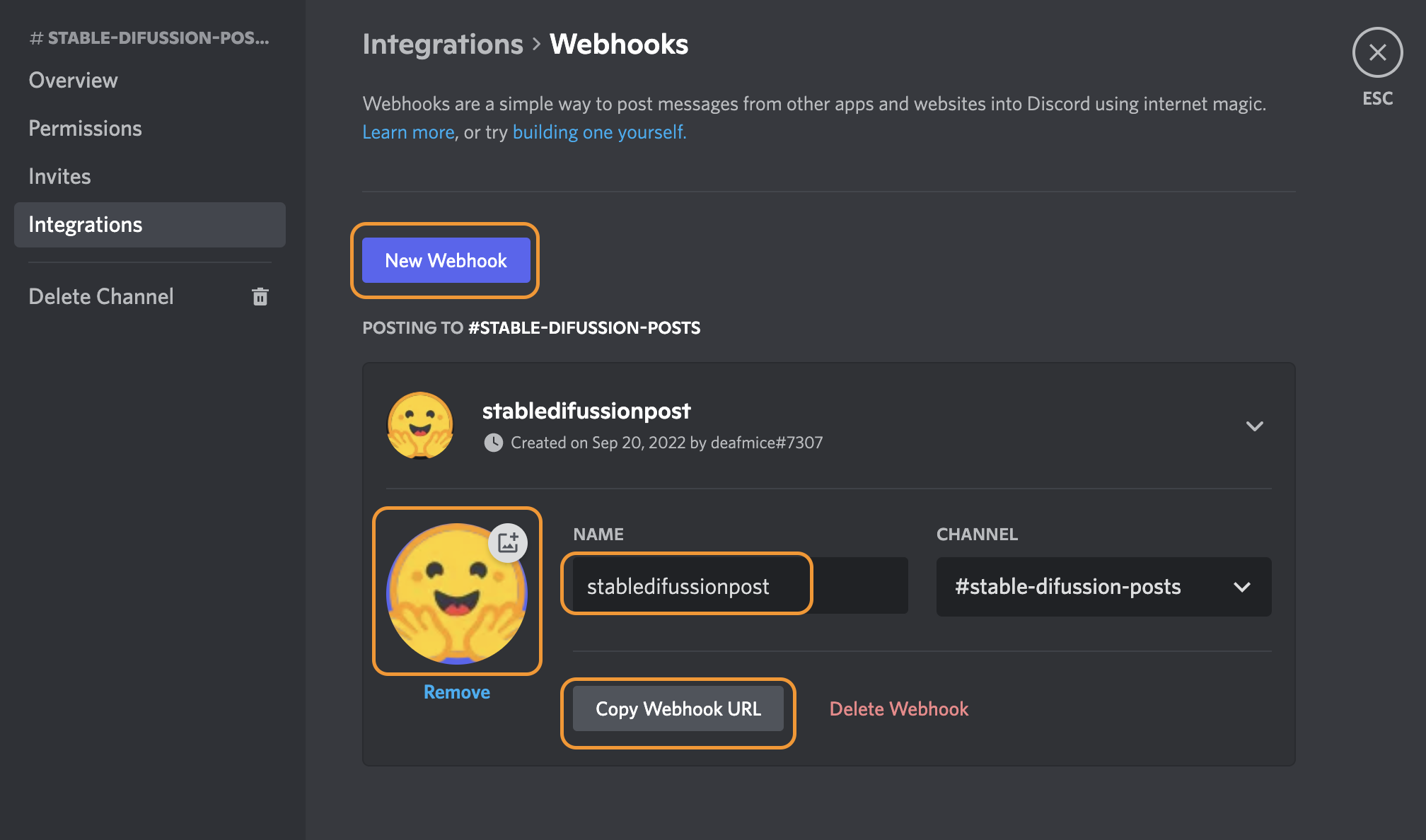
OK, you have the URL, you may want to save it somewhere safe.
Now, let's test it to make sure. Replace YOUR_WEBHOOK_URL with the one from your clipboard.
curl --request POST \
--url YOUR_WEBHOOK_URL \
--header 'Content-Type: application/json' \
--data '{"content": "test"}'
You should see a post in your channel

Some developer docs for future reference:
https://discord.com/developers/docs/resources/webhook
https://discord.com/developers/docs/reference#uploading-files
Create a Python script to post data to that webhook
Create a new file called upload2discord.py
in the stable diffusion
scripts directory as we will be calling it from txt2img.py
and img2img.py
later.
import os
import requests
URL = os.getenv('DISCORD_URL')
def post_webhook(_outpath, _img_name, _prompt):
""" Takes an image and a text prompt and uploads to Discord"""
message = {'content': f'Art Prompt: {_prompt}'}
img_location = os.path.join(_outpath, _img_name)
with open(img_location, "rb") as openfile:
multipart = {"file": (openfile.name, openfile, "application/octet-stream")}
res = requests.post(url=URL, files=multipart, data=message)
if res.status_code == 200:
print("POSTed to Discord.")
else:
print("OOPS, Discord Upload FAILED")
We need to get our webhook we copied early. In the script, I am grabbing an
environment variable called DISCORD_URL
which you can set like this in the terminal:
export DISCORD_URL=YOUR_WEBHOOK_URL_COPIED_EARLIER
This is just for the function post_webhook()
. It takes an
image and a text prompt and then uploads to Discord. We are using
the multipart form to upload the image.
Integrate the new script with text2img and img2img scripts
The integration in both scripts is the same.
We import the post_webhook()
function.
# Uploads image and prompt to Discord
from upload2discord import post_webhook
We then create an argument for the script to post to Discord:
parser.add_argument(
"--discord",
action='store_true',
help="if enabled, uploads the output image to Discord ",
)
Under the outpath
variable declaration, I created an img_name
variable
for later use.
outpath = opt.outdir
img_name = ''
We then change out the img.save()
in/around lines ~359, depending on your
script and when you pulled it from the repos.
I am explicicetly setting the img_name
, and then putting it in img.save()
img_name = f'grid-{grid_count:04}.png'
img.save(os.path.join(outpath, img_name))
Finally at the end, we have a if
statement to check if the user
would like the script to post the image to Discord.
if opt.discord:
# Post the image and the prompt to Discord
post_webhook(outpath, img_name, prompt)
If everything is set, we should get an image posted after Stable Diffusion is done working.
python scripts/txt2img.py \
--prompt "Vinyl Toy, Mouse european explorer in brown+white+black, octane render, chibi body, pink eyes, white fur, 8k" \
--ddim_steps 50 --n_samples 1 \
--n_iter 1 --plms --discord
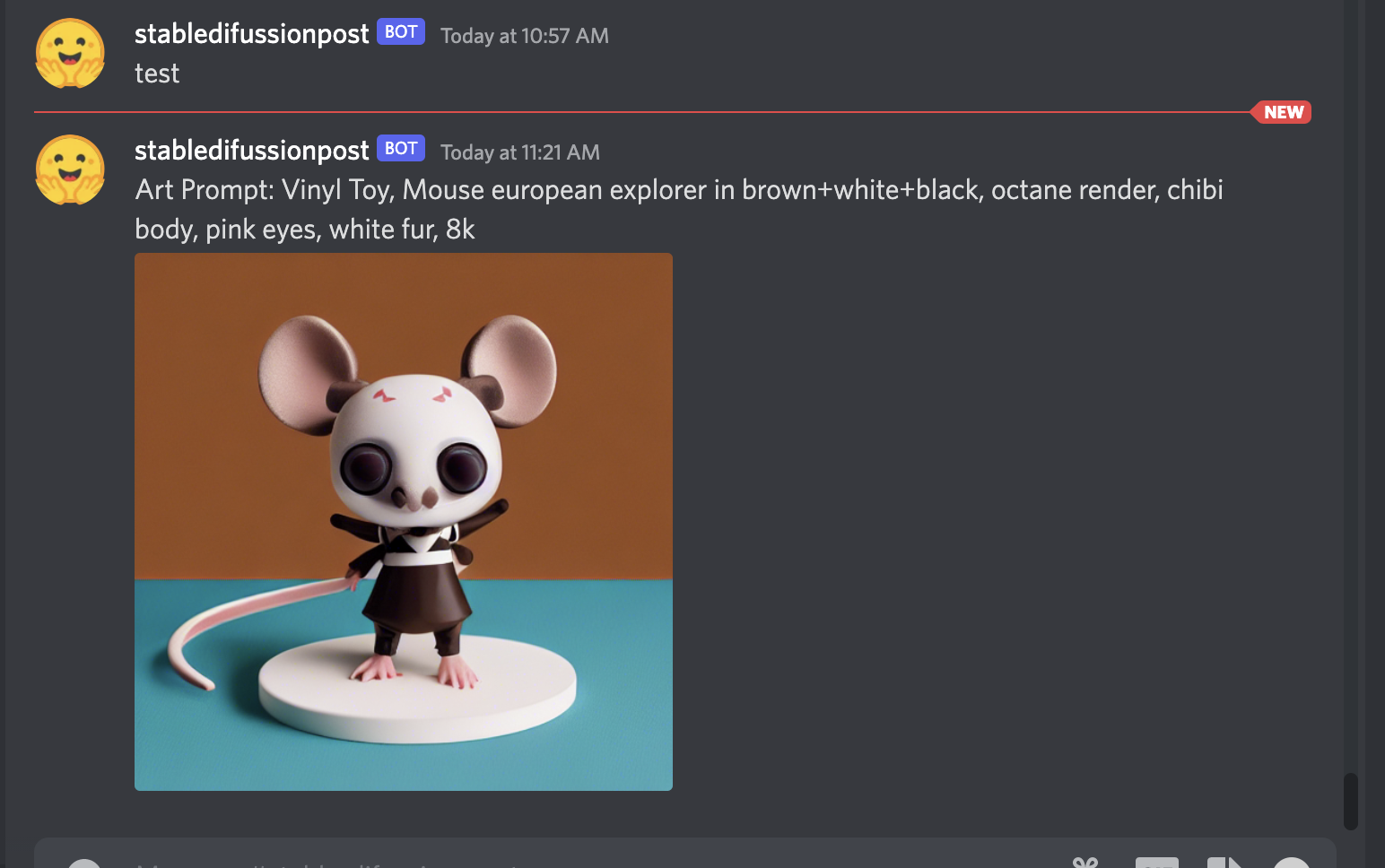
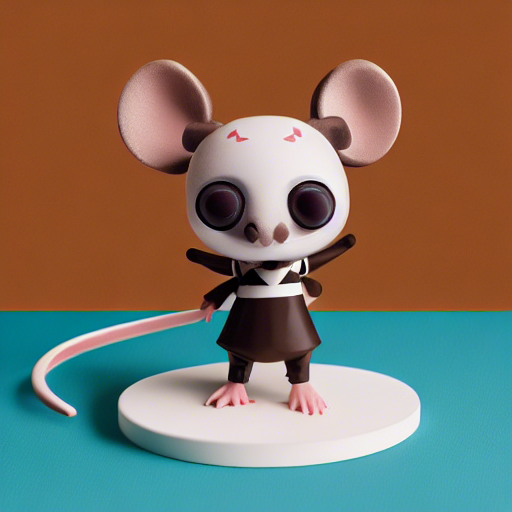
I did the same type of setup in the img2img.py
script.
Final Thoughts
I am really glad that Stable Diffusion uses Python as it gives me the ability to extend my local install to post images to Discord, Telegram.