Building a Futurama Quotes App with Django and CRUD Operations
Learn to build a Futurama Quotes App with Django and CRUD operations. Master web development essentials while creating an engaging app inspired by the beloved animated series. Boost your Django skills with this fun project.
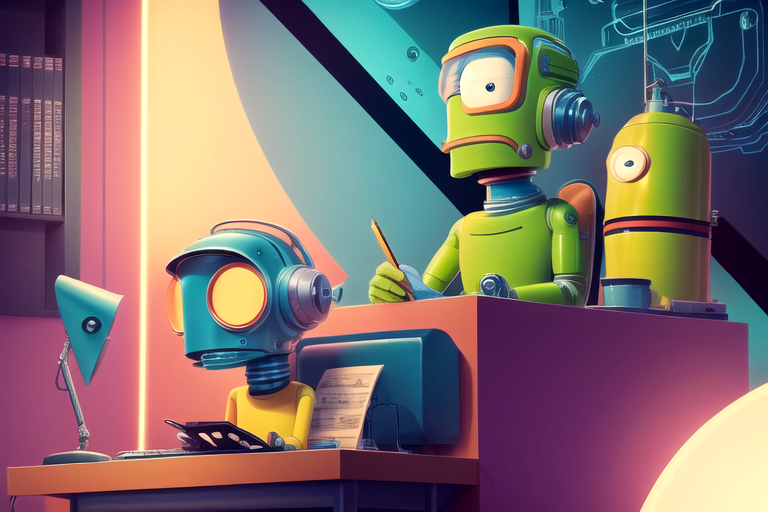
Welcome to another exciting tutorial! Today, we'll be diving into the world of Django, a high-level Python web framework that encourages rapid development and clean, pragmatic design. Our mission is to create a fun and interactive app that allows users to store and manage their favorite quotes from the iconic animated series Futurama.
We'll be implementing a Django app complete with CRUD operations, which stands for Create, Read, Update, and Delete. These are the essential building blocks for any web application that deals with data management. By the end of this tutorial, you'll have a solid understanding of how to create, read, update, and delete data using Django, and how to expose this functionality through a simple REST API.
If you're a fan of Futurama or just eager to learn more about Django and web development, grab your favorite beverage, put on your thinking cap, and let's get started!
Install the required packages:
Django REST Framework documentation
pip install django djangorestframework
Create a new Django project and app:
django-admin startproject futurama_quotes_project
cd futurama_quotes_project
python manage.py startapp futurama_quotes_app
Register the app and the Django REST framework in the futurama_quotes_project/settings.py
file:
INSTALLED_APPS = [
...
'rest_framework',
'futurama_quotes_app',
]
Create the model in futurama_quotes_app/models.py
:
from django.db import models
class Quote(models.Model):
character = models.CharField(max_length=100)
episode = models.CharField(max_length=100)
quote = models.CharField(max_length=500)
def __str__(self):
return f"{self.character} ({self.episode}): {self.quote}"
Create a serializer file in futurama_quotes_app/serializers.py
:
from rest_framework import serializers
from .models import Quote
class QuoteSerializer(serializers.ModelSerializer):
class Meta:
model = Quote
fields = ['id', 'character', 'episode', 'quote']
Create a view in futurama_quotes_app/views.py
:
from rest_framework import generics
from .models import Quote
from .serializers import QuoteSerializer
class QuoteListCreateView(generics.ListCreateAPIView):
queryset = Quote.objects.all()
serializer_class = QuoteSerializer
Create a URL configuration file in futurama_quotes_app/urls.py
:
from django.urls import path
from .views import QuoteListCreateView
urlpatterns = [
path('quotes/', QuoteListCreateView.as_view(), name='quote-list-create'),
]
Include the app's URL configuration in the project's futurama_quotes_project/urls.py
file:
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('api/', include('futurama_quotes_app.urls')),
]
Run migrations:
python manage.py makemigrations
python manage.py migrate
Start the development server:
python manage.py runserver
Now, the Django app should be running, exposing a simple API at http://localhost:8000/api/quotes/
for listing and creating Futurama quotes. To add, update, or delete quotes, you can extend the views, serializers, and URL configurations accordingly.
To interact with the API, you can use tools like curl
, Postman, or your web browser. You can also create a simple frontend using JavaScript's Fetch API to make requests to your Django backend.
The Wrap-up
If you are curious and would like a deeper look at some of the Python code running The Futurama Quote Machine take a look at my repo here:
On the flip side if you are into Flask, I have a repo here:
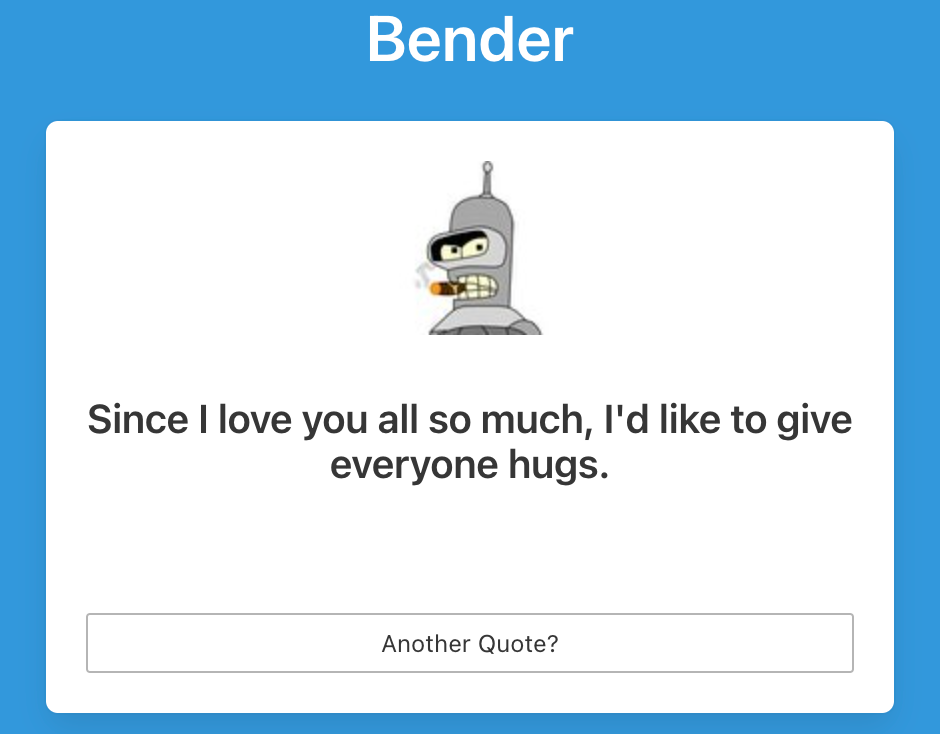
Jeremy
Featured Image Information
Create an illustration of a humorous Futurama-inspired robot character, sitting at a desk and diligently writing an essay. The setting should be whimsical and cartoonish, capturing the essence of the animated series. The robot should exhibit some personality traits reminiscent of Bender, but with a scholarly twist, as it appears to be deeply engaged in composing the blog post.
Negative prompt: (worst quality, low quality:1.4), (logo, text, watermask, username), NG_DeepNegative_V1_75T, (bad-hands-5, bad-artist:1.2)
Steps: 20, Sampler: DPM++ 2M Karras, CFG scale: 7, Seed: 1381895227, Size: 768x512, Model hash: f57b21e57b, Model: revAnimated_v121
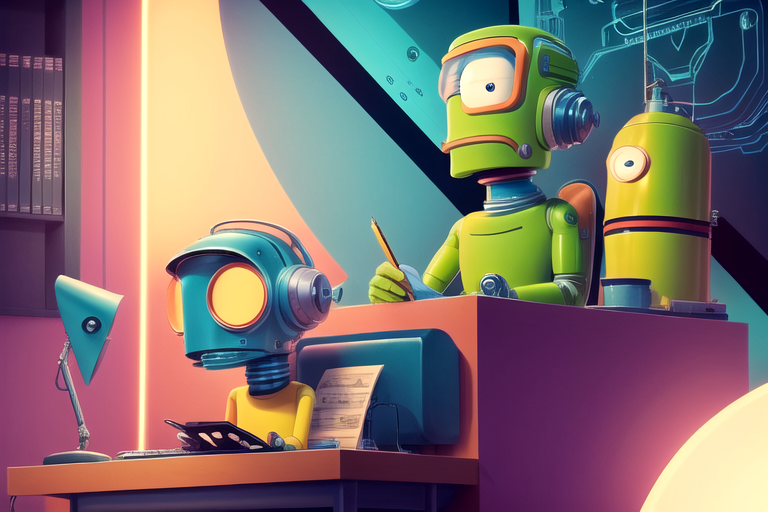